Automating Report Generation with Background Jobs
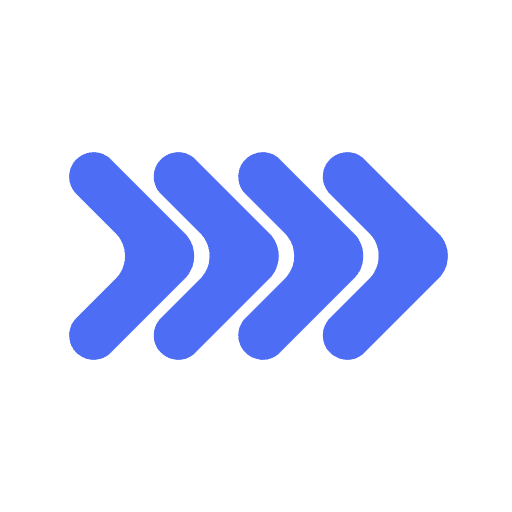
Dispatched Team
1/1/2025
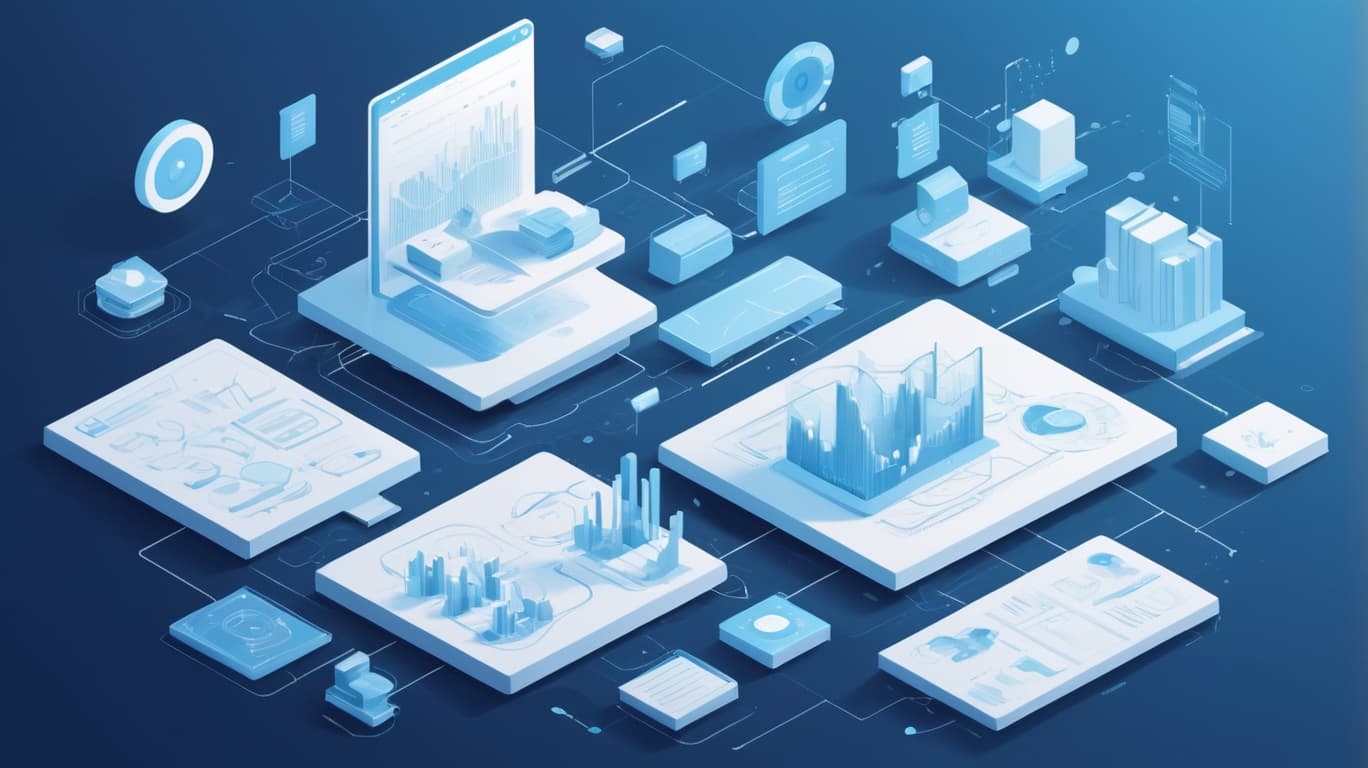
The Challenge with Generating Reports in Real-Time
Generating detailed reports, whether it's analytics, invoices, or summaries, is resource-intensive. For apps that rely on a smooth user experience, tying up server resources to process and deliver reports can slow down other critical tasks and lead to timeouts. This becomes especially problematic as your user base grows.
For example, a content marketing platform might need to generate performance reports for thousands of campaigns. Running this process in real-time could overwhelm your app, leading to delays and frustrated users.
The Solution: Background Jobs
Background jobs offer a scalable way to handle time-consuming tasks like report generation. By queuing these tasks, your app can focus on serving users while the background job processes reports asynchronously. Once the report is ready, it can be delivered via email, a download link, or directly within your app.
Key Benefits of Using Background Jobs for Report Generation:
- Scalability: Handle large workloads without impacting app performance.
- Reliability: Automatically retry failed jobs to ensure delivery.
- User Experience: Keep your app responsive by offloading heavy tasks to the background.
Example Use Case: Marketing Performance Reports
Let’s take the example of a content marketing platform. Here’s how background jobs can streamline the report generation process:
- User Request: A marketer requests a monthly performance report for a specific campaign.
- Queue the Job: Instead of generating the report immediately, the app adds the request to a background job queue.
- Process in the Background: The job fetches data on impressions, clicks, conversions, and ROI, compiles it into a detailed report, and generates a downloadable file.
- Delivery: Once the report is ready, the system sends an email to the marketer with a link to download the report.
This approach ensures that the app remains responsive while efficiently handling report requests.
Step-by-Step Guide: Implementing Background Jobs for Report Generation
Let’s break down how you can implement a background job system for report generation in your app. Here’s a step-by-step guide:
Step 1: Identify the Tasks
Start by identifying which tasks in your app can be offloaded to background jobs. For report generation, this could include:
- Fetching data from a database or analytics API.
- Compiling the data into a specific format (e.g., PDF, Excel).
- Uploading the report to a cloud storage service.
- Notifying the user once the report is ready.
Step 2: Choose a Background Job Tool
Select a tool or service to manage your background jobs. If you’re looking for simplicity and ease of integration, Dispatched is a great choice. It allows you to queue and process jobs using a simple HTTP API.
Step 3: Queue the Job
When a user requests a report, add the job to the queue instead of processing it immediately. Here’s an example of how you can queue a job using Dispatched:
fetch('https://dispatched.dev/api/jobs/dispatch', {
method: 'POST',
headers: {
'Content-Type': 'application/json',
'Authorization': 'Bearer YOUR_API_KEY'
},
body: JSON.stringify({
payload: {
campaignId: '67890',
reportType: 'performance',
month: '2024-11',
action: 'reportGeneration'
}
})
});
Step 4: Process the Job with Webhooks in Next.js (App Router)
Dispatched uses webhooks to process jobs. Here’s how you can handle a webhook in a Next.js App Router setup. The webhook payload includes an action
field to determine how the job should be processed:
// app/api/webhook/route.ts
import { NextResponse } from 'next/server';
export async function POST(req: Request) {
try {
const { action, payload } = await req.json();
switch (action) {
case 'reportGeneration':
// Handle report generation
const report = await generateReport(payload);
await uploadToStorage(report);
break;
case 'reportNotification':
// Handle user notification
await sendNotification(payload);
break;
default:
console.error('Unknown action:', action);
return NextResponse.json({ success: false, error: 'Unknown action' }, { status: 400 });
}
return NextResponse.json({ success: true });
} catch (error) {
console.error('Error processing webhook:', error);
return NextResponse.json({ success: false, error: 'Internal Server Error' }, { status: 500 });
}
}
async function generateReport(payload: { campaignId: string; reportType: string; month: string }) {
const { campaignId, reportType, month } = payload;
// Simulate fetching data and generating a report
return `Report for Campaign ${campaignId}, Type: ${reportType}, Month: ${month}`;
}
async function uploadToStorage(report: string) {
// Simulate uploading the report to cloud storage
console.log('Report uploaded:', report);
}
async function sendNotification(payload: { userId: string; email: string }) {
// Simulate sending an email or in-app notification
console.log('Notification sent for:', payload);
}
Step 5: Notify the User
Once the report is generated and stored, notify the user. This can be done via email or an in-app notification with a link to download the report.
How Dispatched Simplifies Background Jobs
With Dispatched, you can set up background job queues with a simple HTTP API. Here's why it’s a great fit for report generation:
- Pure HTTP Simplicity: Queue jobs with a single HTTP request—no complex setup required.
- Smart Retries: Automatically handle retries for failed jobs.
- Flexible Scheduling: Schedule recurring or delayed jobs with ease.
- Webhooks: Using webhooks ensures that your business logic remains contained within your app, rather than being handled by external services like AWS Lambda.
For our marketing example, you could use Dispatched to queue and manage report generation jobs, ensuring they are processed reliably and delivered on time.
Pro Tips for Scalable Report Generation
- Optimize Data Fetching: Minimize the load on your database or analytics API by using indexed queries and caching where possible.
- Monitor Job Performance: Track job execution times and error rates to identify bottlenecks.
- Implement Security Measures: Ensure that generated reports are accessible only to authorized users by using signed URLs or secure authentication mechanisms.
Conclusion
Background jobs are a powerful way to scale your app while maintaining a seamless user experience. By offloading tasks like report generation to a background queue, you can ensure your app stays fast, reliable, and responsive—even as your user base grows.
Tools like Dispatched make it easy to integrate background jobs into your workflow, so you can focus on building great features without worrying about infrastructure.
Start exploring how background jobs can transform your app today!